Microsoft provides a cloud computing platform called Azure, which is a growing collection of integrated services like analytics, database, mobile, networking, storage and web – for moving faster, achieving more and saving money.
Microsoft provides a set of IoT services (referred to as Microsoft Azure IoT services) over its Azure platform to help build IoT applications rapidly. Microsoft recently launched the Azure IoT suite (on Sept 29th, 2015) which offers pre-configured IoT solutions built on Microsoft’s cloud platform and makes it easy to connect devices securely and supports a broad set of protocols. We will discuss the Azure IoT Suite and its capabilities in detail during the course of the chapter
In the first article, we had discussed about a generic Enterprise IoT stack. Following diagram shows how the how components in the Enterprise IoT stack is mapped with Microsoft Azure IoT services.
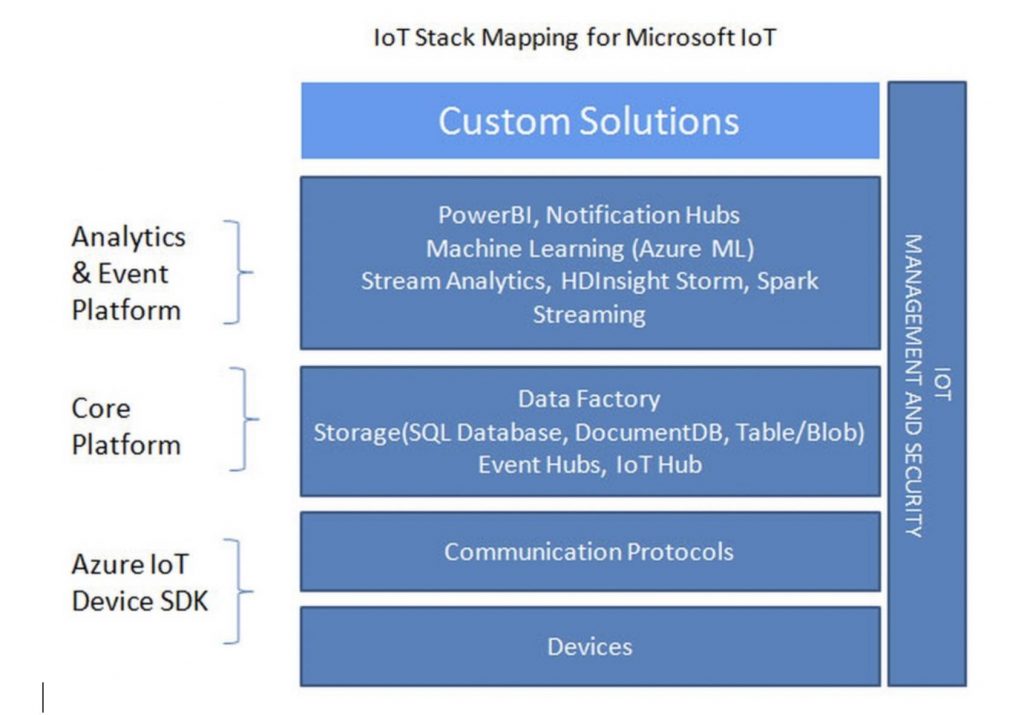
Azure IoT Device SDK
These are device SDK for different platforms like Linux, Windows, and real-time operating systems, which make it easier to get started on any device and connect to Azure IoT platform. These are optional, and a device manufacturer can use the APIs to get started quickly or add its own device connectivity code for connecting to Azure platform e.g. using a JavaScript device API for Intel Edison device or an optimized C SDK for a Linux device. The Azure IoT device SDKs is available on GitHub at https://github.com/Azure/azure-iot-sdks. The Azure IoT SDK connects devices to IoT Hub. We will discuss IoT Hub in detail in the following section.
The following shows a C code snippet which uses the C device SDKS to connect to Azure IoT Hub. The template code can also be generated from the following page: https://azure.microsoft.com/en-us/develop/iot/get-started/
static const char* connectionString ="[device connection string]";
static char msgText[1024];
void main(void){
IoTHUB_CLIENT_HANDLE iotHubClientHandle;
IoTHUB_MESSAGE_HANDLE message;
/* Create IoT Hub Client instance */
iotHubClientHandle = IoTHubClient_CreateFromConnectionString(connectionString, AMQP_Protocol);
/* Setting Message call back so that we can receive Commands. */
IoTHubClient_SetMessageCallback(iotHubClientHandle, ReceiveMessageCallback,&receiveContext);
/* Now that we are ready to receive commands, let's send a message */
sprintf_s(msgText,sizeof(msgText),"{\"deviceId\":\"myFirstDevice\",\"data\":%.2f}", rand()%4+2);
message.messageHandle = IoTHubMessage_CreateFromByteArray((constunsignedchar*)msgText, strlen(msgText));
IoTHubClient_SendEventAsync(iotHubClientHandle, message, SendConfirmationCallback,&message);
/* Add your code here ... */
/* When everything is done, and the app is closing, clean up resources */
IoTHubClient_Destroy(iotHubClientHandle);
}
static IoTHUBMESSAGE_DISPOSITION_RESULT ReceiveMessageCallback(IoTHUB_MESSAGE_HANDLE message,void* userContextCallback)
{
const char* buffer;
size_t size;
IoTHubMessage_GetByteArray(message,(constunsignedchar**)&buffer,&size);
(void)printf("Received Message with Data: <<<%.*s>>>& Size=%d\r\n",(int)size, buffer,(int)size);
/* Some device specific action code goes here... */
return IoTHUBMESSAGE_ACCEPTED;
}
static void SendConfirmationCallback(IoTHUB_CLIENT_CONFIRMATION_RESULT result,void* userContextCallback)
{
IoTHUB_MESSAGE_HANDLE* message =(IoTHUB_MESSAGE_HANDLE*)userContextCallback;
(void)printf("Confirmation received for message tracking id = %d with result = %s\r\n", eventInstance->messageTrackingId, ENUM_TO_STRING(IoTHUB_CLIENT_CONFIRMATION_RESULT, result));
/* Some device specific action code goes here... */
IoTHubMessage_Destroy(*message);
}
Tip – ConnectTheDots.io is another open source project created by Microsoft that allows devices to connect to Azure platform. It was developed before Azure device SDKs and does not use the SDK. Currently, it provides connectivity to Event Hubs only, which we would discuss later
Azure IoT Hub
Azure IoT Hub, a part of the recently released Azure IoT suite, is a fully managed bi-directional device to cloud connectivity bus, providing device management, device security and identity and MQTT protocol support apart from HTTP/AMQP.
Azure IoT Hub provides complete device access through the use of its device identity registry. It provides per device authentication and secures bi-direction connectivity between devices and IoT Hub. The IoT Hub can also integrate with your custom device registry through the use of a token service to create a device-scoped token for secure communication with IoT Hub.
Each Azure IoT Hub allows a certain number of devices which can be simultaneously connected along with the frequency of message that can be transmitted per day by the devices. Scaling Azure IoT Hub is a matter of adding additional IoT Hub units. Azure IoT Hub is capable of handling millions of simultaneously connected devices and millions of events per seconds. For details on IoT Hub units and pricing, kindly refer to this link – https://azure.microsoft.com/en-us/pricing/details/iot-hub/.
Azure IoT Hub classifies the bi-direction messaging capability as device-to-cloud and cloud-to-device messaging. The IoT Hub implements ‘at least once’ delivery guarantees for both device-to-cloud and cloud-to-device messaging. It provides local storage of messages for up to 7 days for device-to-cloud messages and a dedicated device queue for each connected devices for storing cloud-to-device messages that are consumed by the devices securely. This eliminates the overhead of creating separate queues for sending messages back to devices.
Azure IoT Hub and rest of the Azure services parameters can be configured through the Azure Portal.
Event Hubs
Event Hubs is a highly scalable publish-subscribe event processing service. Event Hubs is a part of Azure Service Bus platform that is capable of collecting millions of messages per second with low latency and high reliability, which can be stored for further analysis (historical analysis by machine learning models, auditing, batch processing, etc.) or acted upon directly by stream analytics services. Event Hubs support HTTP and AMQP protocol. Before the release of IoT Hub, Event Hubs was used primarily to support IoT applications. However, Event Hubs only support connecting devices to cloud and does not support per device secure identity out of the box, like the IoT Hub. The Azure device SDKs that we talked about earlier makes it easier to connect to a variety of device platforms and supports connectivity to IoT Hub only, while device connecting to Event Hubs needs to rely on AMQP and HTTP interfaces or use the open source project ConnectTheDots.io. IoT Hub is specifically designed to handle IoT use cases, taking into account device connectivity, identity and secure bi-direction communication between devices and IoT Hub. It is therefore recommended to use IoT Hub for IoT applications. In a typical enterprise IoT application, you would use IoT Hub and Event Hubs in conjunction, where IoT Hub would handle secure device connectivity and handoff messages to Event Hubs for real-time processing.
Storage
The storage comprises of using various options for storing the continuous streams of data. The storage options include SQL Database, DocumentDB (NoSQL) or highly scalable, high-performance Blobs, Tables, and Queues. You also have a choice of using HBase on HDInsight. HDInsight is an Apache Hadoop-based service on Azure cloud. As mentioned earlier, IoT Hub provides the retention of messages for guaranteed delivery, but you would typically use a dedicated storage option to store the messages for further analysis and historical computations. Typically you would use DocumentDB, high-performance Blob or HBase based on volume of data that needs to be stored and analyzed
Azure Data Factory
Azure Data Factory service lets you ingest data from diverse sources (cloud as well as on-premise sources), transform the data and make it consumable for your application. Not all applications may require the use of Data Factory, but we would like to mention this specifically, as your application might need to be integrated with a diverse set of data sources (and even on-premise databases) to correlate or to augment information provided by device data.
Azure Stream Analytics
The Azure Stream Analytics provides real-time stream processing of millions of events per second, enabling you to compare and correlate multiple real-time streams, query data using familiar SQL language and create real-time dashboard and alerts.
Note – The data from Event Hubs can be directly consumed and analyzed by Azure Stream Analytics, without actually storing the data using the storage options.
Microsoft Azure also supports open source streaming solutions like Apache Storm and Apace Spark Streaming as part of the HDInsight services. PaaS vendors should provide support for open source streaming platform solutions and make it easier to implement custom streaming applications without the user worrying about its configuration, scalability or security. One can also look at Azure Data Lake storage service that stores data of any size and allows performing all types of processing and analytics. More details about Azure Data Lake can be found at https://azure.microsoft.com/en-us/solutions/data-lake/.
Machine Learning
Azure Machine Learning provides a visual way to build machine learning model that supports R and Python custom packages, pre-built algorithms and enables us to build a custom model by adding custom code based on the requirements. In future, we envision support for more machine language libraries and expect this to be a marketplace for machine learning models. These learning models will be specifically targeted towards IoT use cases like predictive maintenance for vehicles, refrigerators, etc. and you might have various such variants and pricing based on the optimization and accuracy levels of the machine learning models.
Events and Reporting
These services include sending notifications, like an alert to mobile phones using push notification via the Notification Hubs service or invoking an action using Azure APIs, for instance calling the on-premise application (like a custom workflow exposed as web services) through Logic Apps. Logic Apps lets you automate processes using visual tools and provide connectors for easily integrating disparate data sources from cloud to on-premises. You could also use PowerBI cloud service which allows visualizing and analyzing the data using powerful and flexible dashboards. The Azure Stream Analytics can feed real-time data events into PowerBI, and you can design dashboards that use the data and can be updated at runtime.
For the PowerBI and Azure Stream Analytics integration, while creating the Azure stream job instance you need to select PowerBI as the output of the streaming job and provide the required settings. We would go over the details in the implementation section. For the data to be made available to PowerBI instance, you need to create a query in the Query Tab of the stream job instance, and output of the query would be made available in PowerBI. You can then use the data to build dashboards.
Note – You need to have a Microsoft Power BI account subscription to use it with Azure Stream Analytics.
Custom Solutions
These are an end to end IoT solutions developed using Azure services. Azure also provides bunch of other services that can be used for building IoT solutions apart from Azure IoT services like – the mobile push service to send an alert based on the analysis, the Redis Cache for high throughput and low latency data access or using the Azure APIs for building custom applications to consume the information from Azure Portal. You can find all these services in the product menu option of Azure website at https://azure.microsoft.com/en-us/.
Note- Most of the cloud services mentioned were not specifically designed for handling IoT requirements, like Event Hubs and Azure Stream Analytics, which can be used for other requirements as well like real-time fraud detection, while products like IoT Hub are specially designed to address IoT requirements. We expect more such offerings in future tailored towards realizing IoT use cases.
Implementation Overview
Let’s understand how to use these services, by taking the example of the connected car use case we discussed earlier. The connected car device manufacturer ties it up with Microsoft Azure platform for using its cloud services to realize the various use cases we discussed in connected car section using the Azure platform.
Hardware and Connectivity
The connected car device manufacturer takes care of hardware devices and network provisioning (using GSM module or using internet connectivity via Bluetooth or WI-FI from Smartphones). The device manufacturer provides reliable connectivity and optimum network utilization (2G/3G/4G LTE). To communicate with the Azure platform, the device comes pre-installed with the connectivity code to the Azure platform using the Azure Device SDKs.
The pre-shipped device comes up with the highest level of security, both on the hardware and software side and a set of unique codes (device ids) with ensures only authorized devices can talk to Azure platform. The device manufacturer has provisioned all the devices (as part of its device design and provision step) using Azure Device Management APIs, which are exposed as HTTP REST endpoints. The device manufacturer also implements commands like pause, start, stop, diagnose device which can be controlled through the IoT Hub. The device software uses the Azure Device SDK to transmit the data from the connected car securely to the Azure IoT platform using JSON format over AMQP protocol. The device also provides a display unit which is used to display usage information and communication from Azure IoT platform.
In the next article, we will look at how to implement the IoT solution using Azure IoT Stack.