In my last blog, I wrote about how to generate code to custom cloud architecture diagrams using the Diagrams tool .This is an extension to the previous blog, where I have created a similar code and diagram representation for Google Cloud DevOp process.
Here is the custom diagram representation of AWS devOps process
The process was part of the AWS devOps blogs
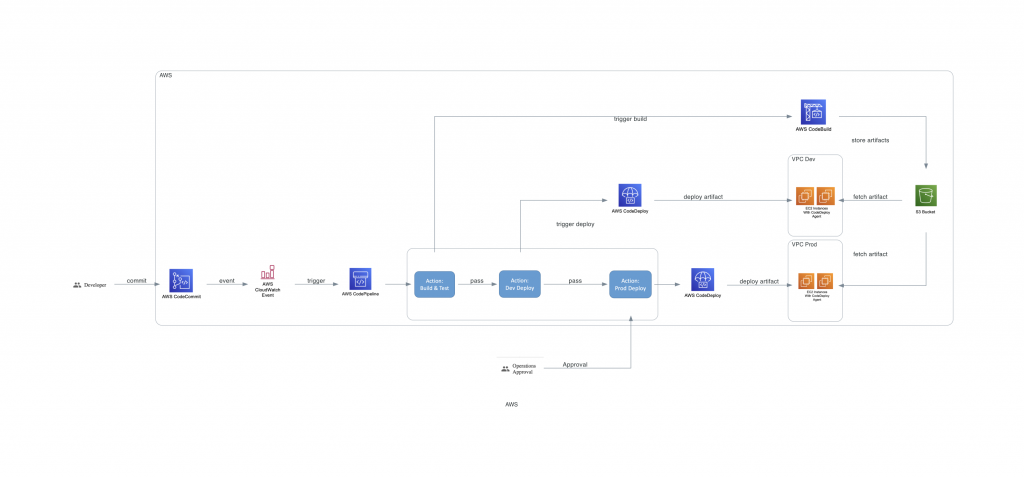
The code to generate the above diagram is given below
from diagrams import Cluster, Diagram, Edge
from diagrams.custom import Custom
graph_attr = {
"fontsize": "12",
"bgcolor": "white",
}
with Diagram("AWS", show=True, graph_attr=graph_attr,direction="LR"):
developer = Custom("","./my_aws_extensions/developer.png")
operationsApproval = Custom("","./my_aws_extensions/operations-approval.png")
with Cluster("AWS", graph_attr=graph_attr):
sourceRepo = Custom("","./my_aws_extensions/aws-code-commit.png")
commitEvent = Custom("","./my_aws_extensions/aws-cloud-watch-event.png")
ciBuild = Custom("","./my_aws_extensions/aws-code-build.png")
ciDeploy = Custom("","./my_aws_extensions/aws-cloud-deploy.png")
ciProdDeploy = Custom("","./my_aws_extensions/aws-cloud-deploy.png")
buildArtifact = Custom("","./my_aws_extensions/aws-s3-build-artifact.png")
pipeline = Custom("","./my_aws_extensions/aws-code-pipeline.png")
sourceRepo >> Edge(label="event") >> commitEvent
commitEvent >> Edge(label="trigger") >> pipeline
#with Cluster("AWS Pipeline", graph_attr=graph_attr):
with Cluster("", graph_attr=graph_attr,direction="TB"):
customBuildTest = Custom("","./my_aws_extensions/custom-build-test.png")
devDeploy = Custom("","./my_aws_extensions/custom-dev-deploy.png")
prodDeploy = Custom("","./my_aws_extensions/custom-prod-deploy.png")
customBuildTest >> Edge(label="pass") >> devDeploy
devDeploy >> Edge(label="pass") >> prodDeploy
pipeline >> customBuildTest
customBuildTest >> Edge(label="trigger build") >> ciBuild
ciBuild >> Edge(label="store artifacts") >> buildArtifact
with Cluster("VPC Dev", graph_attr=graph_attr):
stagingCluster = Custom("","./my_aws_extensions/stage-cluster.png")
with Cluster("VPC Prod", graph_attr=graph_attr):
productionCluster = Custom("","./my_aws_extensions/production-cluster.png")
stagingCluster << Edge(label="fetch artifact") << buildArtifact
devDeploy >> Edge(label="trigger deploy") >> ciDeploy
ciDeploy >> Edge(label="deploy artifact") >> stagingCluster
prodDeploy >> ciProdDeploy
ciProdDeploy >> Edge(label="deploy artifact") >> productionCluster
productionCluster << Edge(label="fetch artifact") << buildArtifact
operationsApproval >> Edge(label="Approval") >> prodDeploy
developer >> Edge(label="commit") >> sourceRepo