In this article, we will introduce a permissionless blockchain implementation called Ethereum. The chapter will cover the core features of Ethereum platform, how to setup a local instance of Ethereum, deploy smart contracts and build an end-to-end sample application.
As per the Ethereum website –
“Ethereum is a decentralized platform that runs smart contracts, applications that run exactly as programmed without the possibility of downtime, censorship, fraud or third party interference.”
Let’s understand this definition in a detail by understanding the terminology and core features of an Ethereum platform.
Core Features of Ethereum platform
In this section, we will talk about the core features of the Ethereum platform.
Application
An application in an Ethereum platform is referred to as a DApp (decentralized application). The DApp typically comprises of a frontend and a backend code. The frontend code is developed using programming languages like JavaScript. The frontend code communicates with the backend code on the blockchain network. The backend code is referred to as a smart contract and is typically developed using a higher-level language like Solidity. The smart contract runs on the decentralized Ethereum network. The DApp maps to the application layer of our reference architecture.
Note – The frontend part of the DApp does not run on Ethereum network. So in an Ethereum context, a DApp could be simply referred to as the smart contract running in the Ethereum network.
Smart Contract
We had described smart contract earlier in Chapter 1. Smart Contract defines and executes transactions in the form of a software code. It comprises of operations that act on the state of the blockchain.
This smart contract acts as a digital contract, which can be used to move value and ownership of assets between parties in a given network or across networks. These contracts can be between a consumer to consumer – like a wire transfer between individuals using their cell phones without any intermediary, a business to business – like a trade finance contract where multiple parties are involved viz. exporter bank, importer bank and shipment vendor, a business to consumer – like an insurance or a health contract which rewards people for their good health behaviors or even between system to system – which enables system to work in a secure and autonomous way, for instance, a contract that defines whether a new device can connect seamlessly to your connected home.
The smart contract in an Ethereum network runs inside an Ethereum Virtual Machine (EVM) and its available on every node in the Ethereum network. Every node has the same copy of the smart contract and runs the same code/logic. We would go through the process of developing and deploying a smart contract during the course of this chapter.
The Smart contract maps to the smart contract layer of our reference architecture.
Note – Solidity is compiled into bytecode that is executed on EVM.
Ether and Gas
To run applications on the public Ethereum blockchain network, you need ethers. Ether is a form of payment – a digital fuel that client applications need to pay in order to deploy the smart contract and execute its operations. Each operation requires some computation; this computation work is referred to as a gas. Different operations require different gas units, which is auto calculated by the system. Based on the gas required, the client needs to pay the appropriate ethers. Think of ether being paid for a gas, as a software service fee for executing the code on the network.
Note – Ether is a form of digital currency, similar to Bitcoin. But instead of using ether as a market currency, the design of Ethereum platform uses ether as a means to run the decentralized applications.
Ethereum Wallet
The Ethereum Wallet allows you to hold and secure ethers and other crypto-assets created on Ethereum. It also allows you to write, deploy and use smart contracts. We would soon see Ethereum Wallet in action as part of our sample application demonstration. The Ethereum Wallet can be mapped to the application layer of our reference architecture, which provides a capability to interact with our Ethereum network.
One important point is to understand how consensus is reached across the Ethereum network and data (i.e., blocks) committed to Ethereum. In the next section, we will talk about this in detail.
Consensus Algorithm in Ethereum
We briefly discussed in chapter 1, different consensus algorithms like Proof-of-Work (PoW), Proof-of-Stake (PoS) and Practical Byzantine Fault Tolerance (PBFT). Ethereum currently uses the PoW algorithm and the plan is to move to PoS in a later release. Let’s understand the PoW consensus algorithm in more detail and what problem it is trying to solve in a decentralized public network.
Ethereum blockchain uses permissionless consensus. Permissionless here means that any node can join and participate in the network and execute or validate the transactions. Out of all the public nodes in the network, majority of the nodes, say 51% out of 100 must agree on the validity of the transaction to arrive at a consensus. Once consensus is reached, the transaction is committed to Ethereum. Now, in the absence of a central governing authority, how can one assure that 51% of these nodes are genuine and no one has added or manipulated nodes to drive transaction in their favor. That’s where the PoW algorithm is applied to drive consensus throughout the network.
The following points explains the PoW algorithm in simple terms
- In a PoW based Ethereum network, each node needs to solve a mathematical puzzle to propose their transaction or to be more precise, an intent to create and commit the block. To solve this mathematical puzzle, it requires lot of computation, power (energy) and time in terms of CPU resources. The nodes solving this puzzle are referred to as miners.
- The mathematical puzzle is all about finding a message digest based on input message + the digest of the previous block.
Note – A message digest is a cryptographic hash function containing a set of digits created by a one-way hashing formula. Digest basically ensures data is not altered and the integrity of the message is maintained between the sender and receiver.
- The mathematical puzzle should be less than the difficulty level of the system. The difficulty level is an arithmetically derived number set by the system. For more details, refer to the example below.
- Once the puzzle is solved, all other nodes should agree to the solution and apply the same formula to arrive at a consensus.
- The node that is the first to solve the mathematical puzzle, and eventually verified and agreed by all other nodes is rewarded for its work. In the Ethereum network, this would imply a node being rewarded with some ethers for solving the puzzle.
Given below is an example of PoW algorithm.
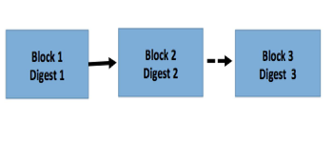
Let’s assume there is a chain of 2 blocks already committed on the network. A new block 3 needs to be added to the existing chain. Let’s understand the sequence of flow as to how this third block of transaction would be committed:
Every node in the network will take up the challenge of creating the third block and committing the same to the blockchain. For this to achieve, each of the nodes will try to solve a mathematical equation. In the context of Ethereum, it is a digest value that needs to be created and it has to be less than some difficulty level preset by the system. The mathematical equation could be something like the following:
Problem to solve: Calculate digest (3) which should be < (less than) the difficulty level
The equation and its context:
Let’s assume the difficulty level is preset as 000000000000000….. 781. Now the digest (3) is calculated using hash algorithm (say sha256) and by creating the hash of the values: block 2, digest 2 and nonce
Note: Nonce can be any arbitrary value that is incremented every time the node makes an attempt to find the correct digest(3) value. In short, nonce is incremented to find a solution to the equation.
Overview of the solution:
Now, each node would start solving this equation by incrementing the nonce value. Let’s say B3 is the new block to be committed, B2 is the previous block i.e. block 2 and D is the difficulty level. If equation is solved the result is Yes, else it is No. The following snippet shows how a node tries to solve the equation:
- Iteration 1 – Sha256 (Block 3, Block 2, 1) < D Outcome – No
- Iteration 2 – Sha256 (Block 3, Block 2, 2) < D Outcome – No
…
- Iteration 2000008 – Sha256 (Block 3, Block 2, 4000008) < D Outcome – Yes
The miner node will keep trying until the outcome is Yes. It is like a rat race where every node attempts to be the first to get the outcome as ‘Yes’. As you can see from the above snippet, at nonce value 4000008, the equation is solved, i.e., the digest(3) value is less than the difficulty level. The node that solves this equation propagates the nonce value of 4000008 to all the other nodes in the network for verification. All other nodes would simply take this nonce value and verify if it is indeed less than the difficulty level. The other nodes just need one-step to verify this equation with least computing power.
Once verified, the block will get committed, i.e., chained to the previous block and the miner is rewarded with ethers for the work done.
Note – As you can see in above example, the blockchain is a chain of hashes, each linked to previous one, i.e., Digest of 3 contains Digest 2, Digest of 2 contains Digest 1 and so on. Adding a new block in between by an intruder implies changing the entire hash sequence till the very beginning, which seems virtually impossible.
In the next part of the article, we will build a crowdsourcing application using an Ethereum platform.